State Management in Flutter Using the Bloc State Manager
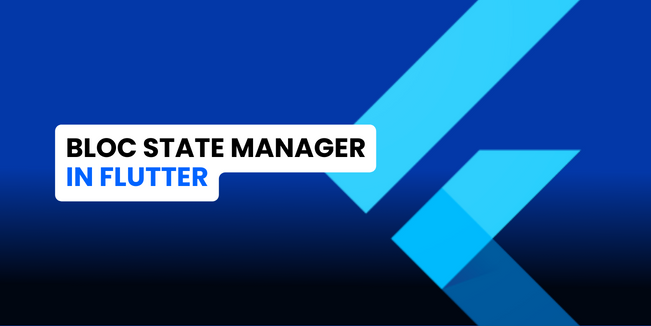
State management is a crucial aspect of developing robust and maintainable Flutter apps. The Bloc pattern offers a powerful, efficient, and flexible solution for handling state in your applications, making it an ideal bloc state manager. In this blog post, we will explore the Bloc pattern, learn how to implement it in a Flutter app, and discuss the advantages it brings to state management. Get ready to unlock the full potential of Bloc and build beautiful, scalable apps with ease!
Key Takeaways
-
The Bloc pattern is an efficient state management solution for Flutter apps, offering clear division of responsibilities and improved UI response.
-
Integrating the Bloc pattern with a UI requires using specific widgets to create a reactive user experience that responds to changes in app states.
-
The Flutter community provides resources such as libraries, tutorials and forums to help developers learn about implementing the Bloc pattern in their projects.
Understanding Bloc State Management
A popular state management solution, the Bloc pattern, distinguishes clearly between business logic and UI code for easy widget tree management in Flutter. The core components of the Bloc pattern are events, Bloc components (business logic components), and states. By streamlining the flow of data between these components, the Bloc pattern allows for easier management of state in Flutter applications.
With a clear division of responsibilities, the Bloc pattern is a remarkable option that offers comprehensible, extensible, and verifiable code for state management in Flutter applications. This pattern enables developers to write less boilerplate code and focus on creating beautiful and functional apps instead.
Events
Events are an integral part of the Bloc pattern, serving as triggers that initiate Bloc functions. They may come from various sources, such as UI widgets or user actions. Events are pivotal in shaping the application’s state since they are processed by the Bloc class to generate a new state.
Applying events in the Bloc pattern guarantees a responsive, event-driven state management approach, leading to an efficient and predictable UI reaction to diverse user interactions and state alterations.
Bloc Components
Bloc components act as intermediaries between events and states, managing business logic for input events and state outputs. The Bloc class processes events and responds with the corresponding state update, allowing for a clear separation of concerns that makes the code easier to maintain and test.
Creating a Bloc class requires extending the Bloc class and implementing the mapEventToState and initialState methods. This structure ensures that the business logic is well-encapsulated within the Bloc class, preventing code duplication and promoting code reusability.
States
States in Flutter represent the information that is processed by any widget. Bloc State Management emphasizes the separation of business logic from presentation and utilizes events and streams to manage state in Flutter apps. This approach facilitates a reactive and testable code structure, as the UI can be adjusted in response to changes in the state.
The use of states in the Bloc pattern, a popular state management pattern, enables developers to build responsive and maintainable UIs that can easily adapt to state changes, enhancing the overall user experience and app performance.
Implementing Bloc in Flutter Apps
Having gained a comprehensive understanding of the Bloc pattern and its components, it’s time to explore its implementation in a Flutter app. We will go through a step-by-step guide on how to add the Bloc library to a Flutter project, create a Bloc class to handle events and manage states, and integrate the Bloc with the UI.
Adhering to these steps in the app development process, as recommended by the flutter team, will equip you to exploit the Bloc pattern’s potential, facilitating the development of sturdy and scalable Flutter apps using the Flutter framework.
Adding Bloc Library
The first step in incorporating the Bloc pattern into your Flutter project is to add the Bloc library. To do so, follow these steps:
-
Open your project in your preferred code editor.
-
Locate the pubspec.yaml file.
-
Include the following line under the dependencies section: flutter_bloc: ^8.0.0 (replace 8.0.0 with the most recent version of the Bloc library).
Save the pubspec.yaml file and execute the command flutter pub get in your terminal to acquire the Bloc library and its dependencies. Once this is done, you can import the Bloc package into your Dart file using the following line: import ‘package:flutter_bloc/flutter_bloc.dart’;.
Creating Bloc Class
With the Bloc library added to your project, the next step is to create a Bloc class that handles events and manages states for a specific feature in your app. To create a Bloc class, extend the Bloc class and implement the mapEventToState and initialState methods.
By creating a dedicated Bloc class for each feature, you ensure that the business logic is well-encapsulated and easy to manage. This approach promotes code reusability and helps to keep your application codebase clean and maintainable.
Integrating Bloc with UI
The final step in implementing the Bloc pattern is to connect the Bloc class with your app’s UI. To do this, you can use the BlocProvider and BlocBuilder widgets. BlocProvider is responsible for providing the Bloc instance to the widgets that need it, while the BlocBuilder widget listens for state changes and updates the UI accordingly.
By integrating the Bloc with the UI using BlocProvider and BlocBuilder, you can create a reactive UI that efficiently responds to state changes, allowing for a more seamless user experience and improved app performance.
Advantages of Using Bloc Pattern in Flutter
Using the Bloc pattern for state management in Flutter apps brings several advantages. First and foremost, it promotes a better separation of concerns, as the business logic is kept separate from the UI code. This makes it easier to maintain, test, and extend your application over time.
Another significant advantage of using the Bloc pattern is code reusability. Since the bloc pattern requires the business logic to be encapsulated within the Bloc class, you can easily reuse it across different parts of your application, reducing code duplication and improving overall code quality.
Furthermore, the improved scalability offered by the Bloc pattern ensures that your app can grow and evolve without becoming unmanageable or difficult to maintain.
Comparing Bloc with Other State Management Solutions
Despite the myriad benefits of the Bloc pattern, it’s worth evaluating other available state management solutions in Flutter like Redux, MobX, and Provider. Each solution has its unique advantages and may be better suited for certain use cases.
Redux is a widely-used state container model that guarantees unidirectional data flow, simplifying state changes and facilitating debugging. MobX, on the other hand, employs reactive programming for redux state management, allowing for efficient state changes and easier debugging.
Provider is another popular solution that uses InheritedWidgets to manage state, providing a simple and efficient approach to state management. When creating a new application, using “class myapp extends statelesswidget” can be a starting point. Ultimately, the choice between these solutions depends on your project’s requirements, your team’s familiarity with the patterns, and personal preferences.
Case Study: Building a Real-World App with Bloc
To demonstrate the Bloc pattern’s efficacy, let’s evaluate a real-world application of a to-do list app constructed using Bloc for state management in Flutter. By implementing the Bloc pattern, the app benefits from a clear separation of concerns, making it easier to maintain and scale as the app grows.
The to-do list app demonstrates how the Bloc pattern can effectively handle state management while maintaining a clean and maintainable codebase. By using the Bloc pattern in your own projects, you can reap the benefits of a straightforward and efficient approach to managing state, along with convenient testing and debugging capabilities.
Tips and Best Practices for Bloc State Management
To fully exploit the Bloc pattern, heed the following tips and best practices:
-
Ensure the Bloc class is dedicated to a single feature by keeping the logic inside the Bloc class as simple as possible.
-
Prevent direct mutation of the state by implementing the Bloc pattern to separate the business logic from the UI.
-
Use packages such as Equatable for more efficient state implementation by providing a mechanism to compare old and new states.
Adhering to these best practices will help you utilize the Bloc pattern effectively in your Flutter applications, resulting in cleaner, more maintainable code and improved app performance.
Flutter Community Resources for Bloc
On your path to mastering the Bloc pattern, a plethora of resources from the Flutter community are available to assist in learning and applying it to your projects. Some of these resources include:
Additionally, you can find informative Medium articles, online forums, and communities such as Reddit and FlutterDev to further enhance your understanding of the Bloc pattern. By leveraging these resources, you’ll be well-equipped to harness the full potential of the Bloc pattern in your Flutter app development projects.
Summary
In conclusion, the Bloc pattern is a powerful and efficient state management solution for Flutter apps, offering a clear separation of concerns, code reusability, and improved scalability. By following the steps outlined in this blog post and utilizing the wealth of resources available in the Flutter community, you can master the Bloc pattern and build beautiful, scalable apps with ease. Embrace the power of the Bloc pattern and elevate your Flutter app development skills today!
Frequently Asked Questions
What is BLoC state management?
BLoC State Management is a pattern which separates business logic from presentation, allowing for reactive and testable code. It utilizes events, streams, and sinks to manage user actions and update the application state.
Why do we use BLoC as state management?
We use BLoC as a state management library because it simplifies complex state management in a clean and organized way, provides centralized state management to track and update the state of the app, and ensures that the user interface is always in sync with the business logic.
Is BLoC the best state management for Flutter?
BLoC is one of the most popular state management libraries for Flutter due to its ability to simplify complex states in a clean and organized way. Although Provider and GetX are good options for smaller apps, BLoC/Cubit, Riverpod, and MobX are better suited for larger apps with more complex states. Ultimately, it depends on the use case and the complexity of the app.
What is Flutter use for?
Flutter is a mobile app development platform created by Google that enables developers to build web, desktop and cross-platform apps for Android and iOS devices. It uses the reactive programming language Dart and works with existing code, allowing for faster and easier development than traditional methods.
What are the core components of the Bloc pattern?
The Bloc pattern is composed of events, business logic components and states, forming a consistent architecture to handle application data flow.