Web Development
Technologies
development
reactnative
reactjs
framework
open-source
How does ReactJs work?
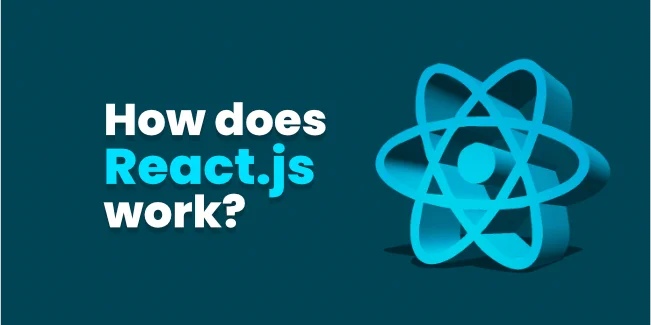
ReactJS, created by Facebook, is a JavaScript library that enables developers to create reusable code snippets for user interface (UI) building called components. It is most popularly used for mobile and web application development.
React is not a JavaScript framework, even though it uses much of the same syntax as frameworks such as Angular and Vue. React is an alternative to frameworks like Angular and Vue, which are all capable of generating sophisticated code.
This article will teach you about React's features, how it works, and its benefits for front-end developers. In addition, we'll discuss the differences between ReactJS and React Native in terms of their functions within the web and mobile app development, respectively.
What is ReactJS?
React is a JavaScript-based UI development framework. Facebook and an open-source community maintain it. Despite the fact that React is a library rather than a language, it is widely utilized in web application development. The software was initially launched in May 2013 and is now one of the most popular frontend libraries for website creation.
Beyond simple user interfaces, React offers extensions for whole application architectural support, such as Flux and React Native.
Features of React
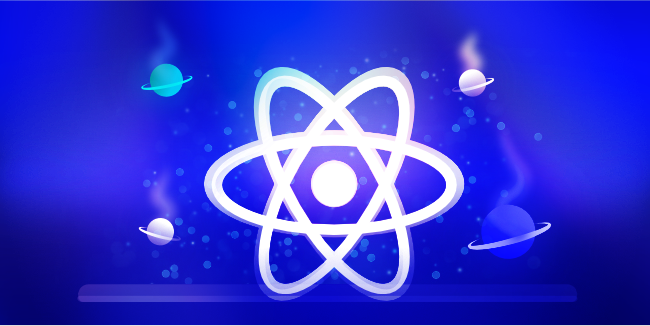
ReactJS has many great features that distinguish it from other JavaScript libraries. The following sections will cover these in-depth and illustrate how they help develop mobile and web applications.
Virtual DOM
The Document Object Model (DOM) is a web page data tree representation. ReactJS keeps Virtual DOM trees in memory. As a result, React may apply changes to specific parts of the data tree rather than re-rendering the whole DOM tree, which is more efficient.
Whenever data changes, ReactJS will create a new Virtual DOM tree and compare it to the previous one to find the quickest way to update the actual DOM. It is called diffing.
Ensuring UI changes impact part of the real DOM tree minimizes the time it takes to render the updated version, saving resources. Large projects with a high level of user interaction will significantly profit from this technique.
JSX
JSX is a JavaScript syntax extension used in React element creation. Developers employ it to embed HTML code in JavaScript objects. JSX can simplify complex code structures by accepting valid JavaScript expressions and function embedding. JSX not only helps prevent Cross-Site Scripting (XSS) attacks but also DOM by automatically converting embedded values in JSX to strings. Therefore, third parties cannot inject any additional code unless it is written explicitly in the application.
Components and Props
ReactJS is a JavaScript library that builds highly interactive user interfaces by combining and reusing components. React components work the same way as JavaScript functions, taking input from properties or props.
On the client side, returned React elements influence how the UI appears. Having as many components as you need without sacrificing readability is easy.
State Management
A JavaScript object that represents a component's section is called a state. When a user interacts with the application, the state changes, displaying a new UI in response to the modifications.
The practice of managing React application states is referred to as state management. It involves saving data in third-party state management libraries, which are then used to trigger the re-rendering process when it changes.
React components may communicate and share data through a state management library. Numerous third-party state management libraries are available today, but Redux and Recoil are two of the most popular.
Redux
The Redux state management library has a single repository that keeps the state tree of an application consistent. The library also minimizes data inconsistency by preventing two components from simultaneously changing the application's state.
Redux's architecture enables error reporting for easier troubleshooting and has a rigid code structure that reduces maintenance work. It also supports a large number of add-ons, is compatible with all UI layers, and includes a lot of add-ons.
However, Redux is rather complicated and hence unsuitable for small apps with a single data source.
Recoil
Recoil is a JavaScript state management library that lets you use pure functions called selectors to calculate data from updateable units of the state known as atoms. Multiple components can subscribe to the same atom and thus share a common state.
Unlike other state management libraries, Recoil's use of atoms and selectors prevents duplicate states, making the code simpler and more efficient. Newcomers to state management will find Recoil easier to understand than Redux because its key concepts are much more straightforward.
Programmatic Navigation
Programmatic navigation uses lines of code to elicit an action that redirects a user. For example, login and signup actions programmatically transport users to new pages. React Router allows you to change which component is rendered without the user clicking on a link, providing multiple ways of safely programmatic navigation between components.
Using a Redirect component is the primary method of programmatic navigation with history.push() is another approach.
React Router allows you to change the look of your React applications without depending on links by synchronizing the UI with the URL.
Why React?
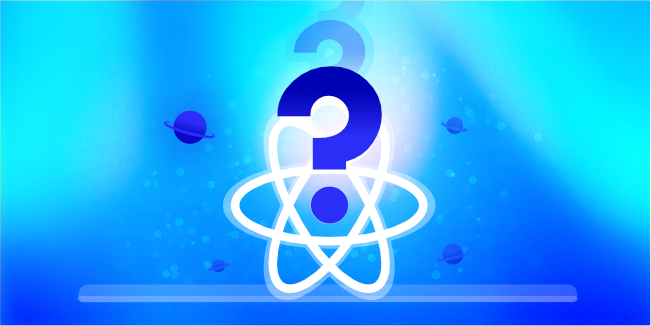
React is used by hundreds of large organizations worldwide, including Netflix, Airbnb, and American Express. In the following sections, we'll explain why many developers choose ReactJS above its competitors.
Easy to Learn
Developers with JavaScript knowledge can learn how to use React in no time as it relies on plain JavaScript and a component-based approach. It's possible to start developing web-based applications with React after just a few days of studying it.
Many sites provide free online tutorials even if you're not a JavaScript expert. Read up on ReactJS to speed up the front-end development process, even if you aren't familiar with JavaScript.
Supports Java Components
ReactJS allows you to recycle components from other applications. ReactJS makes it possible to premade components by being open source, which saves time when creating more complicated web apps.
In addition, React permits nesting components in between others to create complex functions without code expansion. Furthermore, as each component has its own controls, it is effortless to keep up with them.
High-Performance
It's simpler to write React components thanks to JSX integration. Users may use JavaScript combined with HTML typography and tags to construct JavaScript objects. JSX also makes it easier to produce multiple function rendering, which means code is leaner while maintaining the app's capabilities.
JSX may not be the most popular syntax extension, but it has advantages for special components and dynamic application development. React makes it easier to create dynamic web applications by requiring less coding and providing more functionality, as opposed to JavaScript, which can quickly become complicated.
ReactJS's official command-line interface tool, Create React App, simplifies single-page application development. With features like a modern build setup process and pre-configured tools, it is perfect for learning more about ReactJS.
Easier Component Writing
As we mentioned earlier, ReactJS may efficiently update the DOM tree using Virtual DOM. React saves time by memorizing the Virtual DOM in memory, which minimizes wasteful re-rendering and improves performance.
React's one-way data binding between elements helps streamline the debugging process. Any modifications to child components won't affect the parent structure, reducing the risk of errors overall.
SEO Friendly
One of the benefits that ReactJS offers is better SEO for web applications by making them run faster. It is partly due to its implementation of a virtual DOM, which can speed up page loading times.
In addition, React makes it easier for search engines to explore web applications through server-side rendering. It addresses a common problem that websites loaded with JavaScript tend to have since search engines often have difficulty crawling them quickly and effectively.
How Does ReactJS Work?
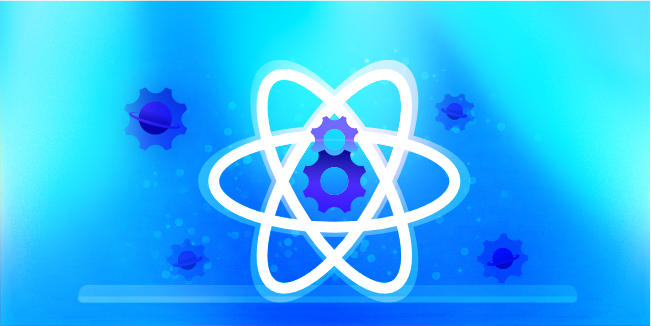
A significant perk of React is that it smoothly merges HTML and JavaScript. In fact, users can generate a DOM node by using the Element function in React.
JSX tags have a name, children, and attributes. Numeric values and expressions must be enclosed in curly brackets. Strings inside JSX attributes are represented by quotation marks, similar to JavaScript strings.
React is most frequently written in JSX rather than regular JavaScript to simplify components and keep the code clean.
We can breakdown the HTML tags as follows:
- MyCounter> count is an example of a variable that represents a numerical expression
- GameScores is an object literal that has two prop-value pairs
- DashboardUnit> is the block of XML that will be displayed on the page
- scores={GameScores} is the scores attribute. The value comes from the GameScores object literal that was defined before.
A React application usually has a single root DOM node. The user interface of a website is modified whenever an element is rendered into the DOM. The Virtual DOM will update the real DOM to match when a React component produces an aspect.
How Does React Native Fit In With It?
Knowing what ReactJS is, you probably heard something about React Native. What is it?
React Native is a free, open-source JavaScript framework based on the React library. It's used to create cross-platform React applications for iOS and Android.
React Native uses native Application Programming Interfaces (APIs) to render mobile UI components in Objective-C (iOS) or Java (Android). As a result, developers may create platform-specific components and share the source code across multiple platforms.
React Native and ReactJS have several characteristics in common, but they are not the same. How does React Native differ from ReactJS in the case of mobile and web development?
ReactJS
- A JavaScript library
- For building dynamic web applications
- Uses Virtual DOM to render browser code
- Supports CSS for animations
- Uses HTML tags
- Uses CSS for styling
React Native
- A JavaScript framework
- Its mobile apps' UI has a native feeling.
- Uses native APIs to render code on mobile devices
- The animated API is necessary to animate components.
- Because DOM support is limited, this script does not utilize HTML tags.
- Uses the JS stylesheet
ReactJS Equals Performance
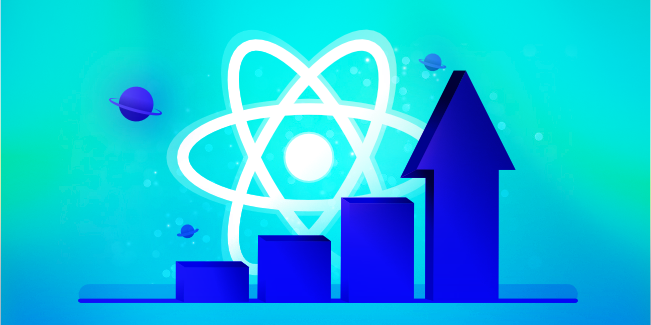
The ability to express the structure of a user interface with JSX makes React a more declarative programming language than imperative. Although this is less error-prone than building the UI one element at a time, it does come with a performance issue.
For example, having a declarative UI structure is fine for the initial rendering because there are no other elements on the page to interfere. So the React renderer can look at JSX and render it into HTML without issues.
When a React component is first rendered, it and its JSX are no different from other template libraries. For example, Handlebars renders a template to HTML markup as a string inserted into the browser DOM. React differs from libraries like Handlebars; we need to re-render the component when data changes.
The result is that the Handlebars constructor function will rebuild the entire HTML string, exactly as it did on the initial render. Because this is inefficient, we frequently resort to imperative workarounds to manually update tiny portions of the DOM. We end up with a tangled tangle of declarative templates and imperative code for dealing with dynamic UI features.
Final Thoughts
ReactJS is a JavaScript library that's particularly useful in creating dynamic web applications. It simplifies JavaScript coding and enhances your application's performance and SEO, among other things.
ReactJS can help simplify the debugging process and reduce errors by only allowing data to flow in one direction.
Here's a summary of the advantages of using React:
- It's simple to use and learn, with plenty of online coding tutorials to help you get started.
- It enables you to develop components that can be reused. It shortens the time it takes to create software.
- The use of JSX makes it simpler to code and render components.
- The reason for re-rendering is eliminated with a virtual DOM, which ensures that your program performs well.
- React helps search engines crawl your site, which improves its SEO.
We hope this article helped you understand what ReactJS is, how it differs from React Native, and why you should use it. If you are looking for someone who will explain the secrets of knowledge about web and mobile development, Contact Us!